Effortless Guide To Sending Objects In URLs: A Comprehensive Overview
How do I send objects in a URL? Ultimately, you can't send objects in a URL. A URL can only contain a string of characters. However, you can send objects as part of the query string or as part of the request body.
The query string is the part of the URL that comes after the question mark (?). The request body is the data that is sent with the request. You can use the query string to send simple data, such as a user ID or a search query. You can use the request body to send more complex data, such as a JSON object.
There are several benefits to sending objects in the query string or request body. First, it allows you to send more data than you could in the URL itself. Second, it keeps the URL clean and readable. Third, it makes it easier to parse the data on the server side.
There are a few things to keep in mind when sending objects in the query string or request body. First, the data must be properly encoded. Second, the server must be able to parse the data. Third, the data should be validated to ensure that it is correct.
How to Send Objects in URL
Sending objects in URL is a common practice in web development. It allows you to pass data from one page to another without having to store it in the database or use cookies.
- Query String: The query string is the part of the URL that comes after the question mark (?). It is used to send simple data, such as a user ID or a search query.
- Request Body: The request body is the data that is sent with the request. It is used to send more complex data, such as a JSON object.
- Encoding: The data in the query string or request body must be properly encoded. This is to ensure that the data is not corrupted during transmission.
- Parsing: The server must be able to parse the data in the query string or request body. This is to ensure that the data can be used by the application.
- Validation: The data in the query string or request body should be validated to ensure that it is correct.
Sending objects in URL is a powerful technique that can be used to improve the functionality and performance of your web applications.
Query String
The query string is an essential part of sending objects in a URL. It allows you to pass data from one page to another without having to store it in the database or use cookies. This can be useful for a variety of purposes, such as:
- Passing user-specific information: You can use the query string to pass user-specific information, such as their user ID or their search query. This information can then be used to personalize the user's experience on the website.
- Tracking website traffic: You can use the query string to track website traffic. For example, you can use the query string to track which pages users are visiting and how long they are staying on each page.
- Passing data between pages: You can use the query string to pass data between pages. For example, you can use the query string to pass the results of a search query from one page to another.
The query string is a powerful tool that can be used to improve the functionality and performance of your website. By understanding how to use the query string, you can make your website more user-friendly and efficient.
Request Body
The request body is an essential part of sending objects in a URL. It allows you to send more complex data than you could in the query string. This is useful for sending data that is too large or complex to fit in the query string, such as a JSON object.
- Data Structure: The request body can contain any type of data, including JSON objects, XML documents, and plain text. This makes it a flexible and powerful way to send data to a server.
- Size Limit: The size of the request body is limited by the server. This limit is typically set to prevent Denial of Service (DoS) attacks.
- Security: The request body is not visible in the URL, which makes it more secure than the query string. This is important for sending sensitive data, such as passwords or credit card numbers.
The request body is a powerful tool that can be used to send complex data to a server. By understanding how to use the request body, you can improve the functionality and performance of your web applications.
Encoding
When sending objects in a URL, it is important to properly encode the data. This is to ensure that the data is not corrupted during transmission. Corrupted data can lead to errors on the server side, and can even make your application vulnerable to attack.
There are a number of different ways to encode data. The most common method is to use percent-encoding. Percent-encoding replaces special characters with their corresponding percent-encoded values. For example, the space character is encoded as "%20".
Here is an example of a properly encoded URL:
https://example.com/user?id=123
In this example, the user ID (123) has been properly encoded using percent-encoding.
It is important to note that not all characters need to be encoded. Only characters that have special meaning in a URL need to be encoded. For example, the following characters do not need to be encoded:
- a-z
- A-Z
- 0-9
- _-
- .
By properly encoding your data, you can ensure that it is transmitted securely and without errors.
Here are some of the benefits of properly encoding data:
- Prevents data corruption
- Protects against attacks
- Improves performance
If you are sending objects in a URL, it is important to understand how to properly encode your data. By doing so, you can ensure that your data is transmitted securely and without errors.
Parsing
Parsing is the process of converting data from one format to another. In the context of sending objects in a URL, parsing is the process of converting the data in the query string or request body into a format that can be used by the application. This is important because the data in the query string or request body is typically in a raw format that is not directly usable by the application.
For example, if the data in the query string is a JSON object, the server must be able to parse the JSON object into a format that can be used by the application. This may involve converting the JSON object into an array, a hash table, or some other data structure that is more easily usable by the application.
Parsing is an essential part of sending objects in a URL. Without parsing, the server would not be able to use the data in the query string or request body. This would make it impossible to send complex data to a server using a URL.
Here are some of the benefits of parsing data:
- Makes data usable by the application
- Improves performance
- Protects against attacks
If you are sending objects in a URL, it is important to understand how to parse data. By doing so, you can ensure that your data is transmitted securely and without errors.
Validation
Validation is an essential part of sending objects in a URL. Without validation, there is no guarantee that the data in the query string or request body is correct. This can lead to errors on the server side, and can even make your application vulnerable to attack.
There are a number of different ways to validate data. The most common method is to use data validation rules. Data validation rules define the expected format and range of values for a particular piece of data. For example, a data validation rule for a user ID might specify that the user ID must be a positive integer.
If the data in the query string or request body does not match the data validation rules, the server should reject the data. This will help to prevent errors and protect your application from attack.
Here are some of the benefits of validating data:
- Prevents errors
- Protects against attacks
- Improves performance
If you are sending objects in a URL, it is important to understand how to validate data. By doing so, you can ensure that your data is transmitted securely and without errors.
Here is an example of how to validate data in a URL:
function validateData(data) { // Check if the data is a valid JSON object try { JSON.parse(data); } catch (e) { return false; } // Check if the data contains the required fields if (!data.hasOwnProperty('name') || !data.hasOwnProperty('age')) { return false; } // Check if the data is valid if (data.name === '' || data.age < 0) { return false; } // The data is valid return true;}
This function can be used to validate data in a query string or request body. If the data is valid, the function will return true. Otherwise, the function will return false.
By validating data, you can ensure that your application is receiving accurate and reliable data. This will help to prevent errors and protect your application from attack.
FAQs on "How to Send Objects in URL"
This section provides answers to frequently asked questions about sending objects in URL.
Question 1: What is the purpose of sending objects in URL?Sending objects in URL allows you to pass data from one page to another without having to store it in the database or use cookies. This can be useful for a variety of purposes, such as passing user-specific information, tracking website traffic, and passing data between pages.
Question 2: What are the different ways to send objects in URL?There are two main ways to send objects in URL: the query string and the request body. The query string is the part of the URL that comes after the question mark (?). It is used to send simple data, such as a user ID or a search query. The request body is the data that is sent with the request. It is used to send more complex data, such as a JSON object.
Question 3: What are the benefits of sending objects in URL?There are several benefits to sending objects in URL. First, it allows you to send more data than you could in the URL itself. Second, it keeps the URL clean and readable. Third, it makes it easier to parse the data on the server side.
Question 4: What are the challenges of sending objects in URL?There are a few challenges to sending objects in URL. First, the data must be properly encoded. Second, the server must be able to parse the data. Third, the data should be validated to ensure that it is correct.
Question 5: What are the best practices for sending objects in URL?There are a few best practices to follow when sending objects in URL. First, use the query string for simple data and the request body for more complex data. Second, properly encode the data to prevent corruption. Third, parse the data on the server side to ensure that it is in a usable format. Fourth, validate the data to ensure that it is correct.
By following these best practices, you can ensure that your objects are sent securely and efficiently in URL.
This concludes the FAQs on "How to Send Objects in URL". If you have any further questions, please consult the documentation or contact support.
Next: Advanced Techniques for Sending Objects in URL
Conclusion
In this article, we have explored how to send objects in URL. We have discussed the different ways to send objects in URL, the benefits and challenges of sending objects in URL, and the best practices for sending objects in URL.
Sending objects in URL is a powerful technique that can be used to improve the functionality and performance of your web applications. By understanding how to send objects in URL, you can make your applications more user-friendly, efficient, and secure.
We encourage you to experiment with sending objects in URL to see how it can benefit your own applications.
How To Easily Uninstall Cylance Protect
Why Does Colic Tend To Be Worse At Night? Causes And Remedies
Forever Unstoppable: Death's Inability To Halt My Journey

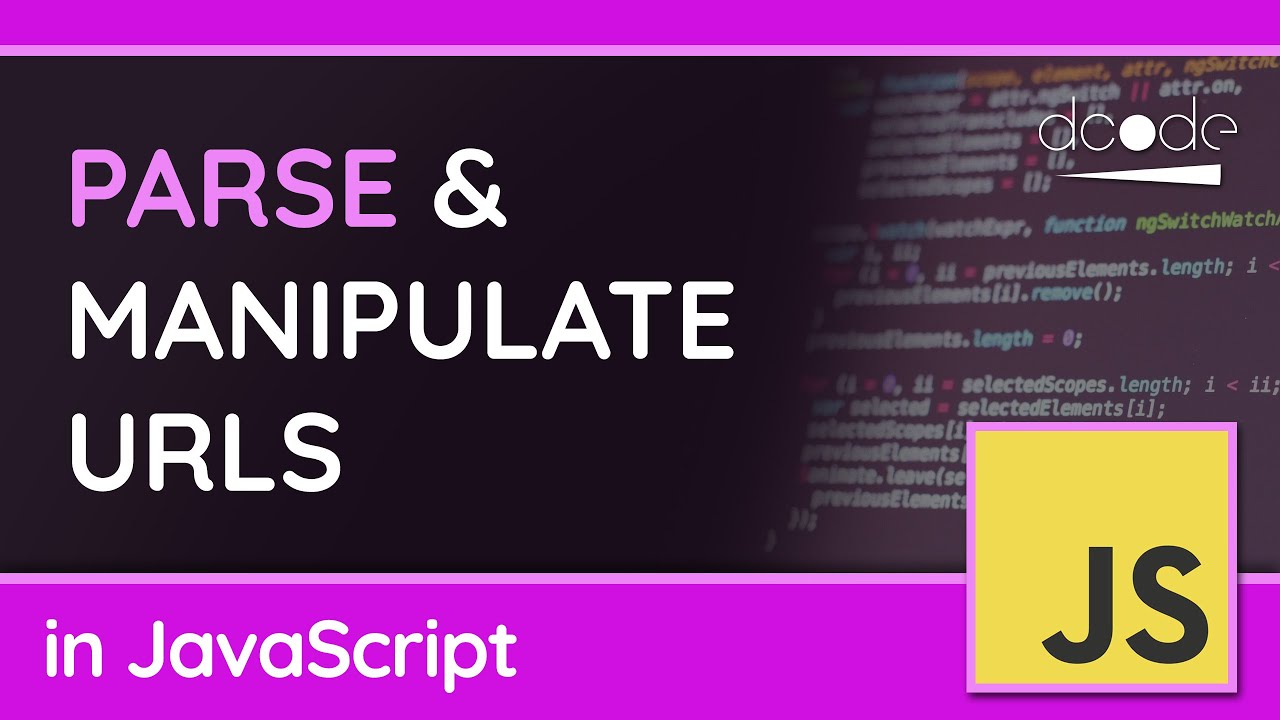
